- Prime 소수
- Composite 복합수
만약 숫자 a가 n의 약수라면, n/a도 n의 약수입니다.
이 두 개의 약수 중 하나가 √n보다 작거나 같습니다.
➔ 1부터 √n까지의 모든 숫자를 반복하면 모든 약수을 찾을 수 있습니다.
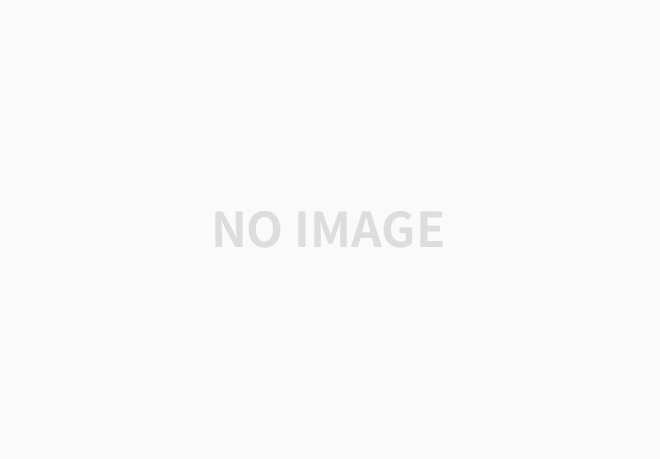
#약수 갯수세기 함수
def divisors(n):
i=1
result=0
while i*i<n: #1부터 √n까지 반복하면 모든 약수을 찾을 수 있음
if n%i==0:
result+=2
i+=1
if i*i==n:
result+=1
return result
def primality(n):
i=2
while i*i<=n:
if n%i==0:
return False
i+=1
return True #나눠지는게 없으면 소수!
10.1 CountFactors
A positive integer D is a factor of a positive integer N if there exists an integer M such that N = D * M. Write a func that, given a positive integer N, returns the number of its factors.
#divisors함수랑 똑같!!!
def solution(N):
i = 1
result = 0
while (i * i < N):
if (N % i == 0):
result += 2
i += 1
if (i * i == N):
result += 1
return result
10.2 MinPerimeterRectangle
An integer N is given, representing the area of some rectangle. The area of a rectangle whose sides are of length A and B is A * B, and the perimeter is 2 * (A + B). The goal is to find the minimal perimeter of any rectangle whose area equals N.
Write a func that, given an integer N, returns the minimal perimeter of any rectangle whose area is exactly equal to N.
어떤 직사각형의 넓이를 나타내는 정수 N이 주어집니다. 변의 길이가 A, B인 직사각형의 넓이는 A * B이고, 둘레는 2 * (A + B)입니다. 목표는 넓이가 N인 직사각형의 최소 둘레를 찾는 것입니다. 정수 N이 주어지면 면적이 N과 같은 직사각형의 최소 둘레를 반환하는 함수를 작성합니다.
def solution(N):
i = 1
result = (N+1)*2 # N*1의 면적도 N이랍니다
while (i * i < N):
if (N % i == 0):
result = min(result, i*2 + (N//i)*2 )
i += 1
if (i * i == N):
result = min(result, i*4 )
return result
'Computer Science > 알고리즘' 카테고리의 다른 글
정렬 알고리즘 간단 정리 (0) | 2023.10.25 |
---|---|
[Codility] 11. Sieve of Eratosthenes (CountNonDivisible) (1) | 2023.10.23 |
[Codility] 9. Maximum slice problem (MaxProfit, MaxSliceSum) (1) | 2023.10.23 |
[Codility] 8. Leader (Dominator, EquiLeader) (1) | 2023.10.23 |
[코드트리 챌린지] 재귀함수 활용 (1) | 2023.10.19 |